- Java Tutorial
- Java Spring
- Spring Interview Questions
- Java SpringBoot
- Spring Boot Interview Questions
- Spring MVC Interview Questions
- Java Hibernate
- Hibernate Interview Questions
- Advance Java Projects
- Java Interview Questions

JPA - ORDER BY Clause
JPA in Java can be defined as the Java Persistence API and consists of Java instructions for accessing and managing data between Java objects and related databases. JPA provides ways for us to access object-oriented and complex databases through SQL queries and database connections. There are many clauses in JPA and the ORDER BY clause is one of them. The ORDER BY clause can be used to sort the query results in ascending or descending order based on the fields.
ORDER BY Clause in JPA
The ORDER BY clause in JPA is used to sort query results generated in a JPQL (Java Persistence Query Language) query. This allows us to format the query results in the desired format and simplifies data interpretation and manipulation.
Steps to Implement ORDER BY Clause:
- Define the JPQL query with the SELECT statement of the JPA application.
- We can append the ORDER BY clause to the query and it can specify the fields by which results should be sorted.
- Execute the query and retrieve the sorted results of the program.
1. Sorting in Ascending Order
In the JPA Application, we can sort the query results in ascending order and it can specify the fields in the ORDER BY clause without the additional keywords.
2. Sorting in Descending Order
We can sort the query results in descending order add the DESC keyword after the field in the ORDER BY clause.
3. Sorting by Multiple Fields
We can sort the query results by the multiple fields by the listing in the ORDER BY clause separated by the commas.
4. Using Entity Relationships for Sorting
Entity relationships such as the one-to-many or many-to-one associations and it can be leveraged for the sorting. It can be performed based on the attributes of the related entities.
Sorting the employees by the name of their department or by date of joining where the department information or the joining date is stored in the related entities.
Step-by-Step Implementation of JPA ORDER BY Clause
We can develop the simple JPA application that can demonstrate the ORDER BY clause of the application.
Step 1 : Create the new JPA project using the Intellj Idea named jpa-order-by-clause-demo. After creating the project, the file structure looks like the below image.
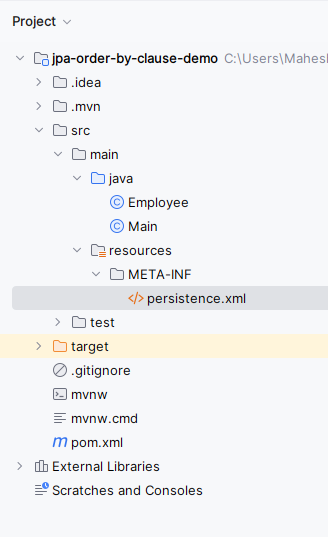
Step 2 : Open the open.xml and add the below dependencies into the project.
Step 3 : Open the persistence.xml and put the below code into the project and it can configure the database of the project.
Step 4 : Create the table in MySQL database using the below SQL query:
Step 5 : Create the new Entity Java class named as the Employee .
Go to src > main > java > Employee and put the below code.
Step 6 : Create the new Java main class named as the Main .
Go to src > main > java > Main and put the below code.
Step 7 : Once the project is completed, run the application. It will then show the Employee name and salary by the descending order as output. Refer the below output image for the better understanding of the concept.
By the following the above steps of the article, developers can gain the soild understanding of the how to effectively use the ORDER BY clause in their JPA applications.
Similar Reads
- JPA - ORDER BY Clause JPA in Java can be defined as the Java Persistence API and consists of Java instructions for accessing and managing data between Java objects and related databases. JPA provides ways for us to access object-oriented and complex databases through SQL queries and database connections. There are many c 6 min read
- MySQL ORDER BY Clause In MySQL, the ORDER BY Clause is used to sort the result set either in ascending order or descending order. By default, the ORDER BY sorts the displayed data in ascending order. If you want your data displayed in descending order we need to use the DESC keyword along with the ORDER BY Clause. To mak 5 min read
- SQLite ORDER BY Clause SQLite is the most popular database engine which is written in C programming language. It is a serverless, easy-to-use relational database system and it is open source and self-contained. In this article, you will gain knowledge on the SQLite ORDER BY clause. By the end of this article, you will get 8 min read
- Python SQLite - ORDER BY Clause In this article, we will discuss ORDER BY clause in SQLite using Python. The ORDER BY statement is a SQL statement that is used to sort the data in either ascending or descending according to one or more columns. By default, ORDER BY sorts the data in ascending order. DESC is used to sort the data i 3 min read
- JPA - Criteria WHERE Clause JPA in Java is defined as the Java Persistence API and the Criteria API provides a structured and type-safe way to build dynamic queries at runtime. The WHERE clause is an integral part of any SQL query and allows us to modify records based on specified conditions. Similarly, the WHERE clause in the 6 min read
- PL/SQL WHERE Clause The WHERE clause in PL/SQL is essential for filtering records based on specified conditions. It is used in SELECT, UPDATE, and DELETE statements to limit the rows affected or retrieved, allowing precise control over data manipulation and retrieval. In this article, We will learn about the WHERE Clau 4 min read
- WHERE Clause in MariaDB MariaDB uses SQL (Structured Query Language) and it is an open-source relational database management system (RDBMS) for managing and manipulating data. The WHERE clause in SQL queries is used to filter and obtain specific data. The ability to remove and retrieve specific data using the WHERE clause 3 min read
- JPA - Criteria GROUP BY Clause JPA in Java can be called the Java Persistence API. It provides the Criteria API as a programming mechanism for creating queries dynamically. One of these important features is the GROUP BY clause which allows developers to group query results based on specific criteria. GROUP BY ClauseThe Group BY 7 min read
- MySQL WHERE Clause The MySQL WHERE clause is essential for filtering data based on specified conditions and returning it in the result set. It is commonly used in SELECT, INSERT, UPDATE, and DELETE statements to work on specific data. This clause follows the FROM clause in a SELECT statement and precedes any ORDER BY 5 min read
- PostgreSQL - WITH Clause The WITH clause in PostgreSQL, also known as a Common Table Expression (CTE), simplifies complex queries by breaking them into smaller, readable sections. It allows us to define temporary result sets that can be referenced later in our main query. This makes the PostgreSQL code easier to manage and 4 min read
- JPA - Criteria SELECT Clause In Java, JPA is defined as the Java Persistence API and the Criteria API provides a systematic way to create queries dynamically. It provides more flexibility and type safety compared to JPQL (Java Persistence Query Language). Criteria SELECT ClauseThe Criteria API in JPA allows developers to constr 5 min read
- How to Custom Sort in SQL ORDER BY Clause? By default SQL ORDER BY sort, the column in ascending order but when the descending order is needed ORDER BY DESC can be used. In case when we need a custom sort then we need to use a CASE statement where we have to mention the priorities to get the column sorted. In this article let us see how we c 2 min read
- SQLite WHERE Clause SQLite is the serverless database engine that is used most widely. It is written in c programming language and it belongs to the embedded database family. In this article, you will be learning about the where clause and functionality of the where clause in SQLite. Where ClauseSQLite WHERE Clause is 3 min read
- SQLite HAVING Clause SQLite is a server-less database engine and it is written in c programming language. The main moto for developing SQLite is to escape from using complex database engines like MySQL etc. It has become one of the most popular database engines as we use it in Television, Mobile Phones, web browsers, an 5 min read
- PL/SQL WITH Clause The PL/SQL WITH clause is a powerful feature that enhances the readability and performance of your SQL queries. It allows you to define temporary result sets, which can be referenced multiple times within a single query. This feature is particularly useful for simplifying complex queries and improvi 5 min read
- SQL - Multiple Column Ordering SQL stands for Structured Query Language. It is used to communicate with the database. There are some standard SQl commands like 'select', 'delete', 'alter' etc. For column ordering in SQL, we use the 'ORDER BY' keyword. Order By:It is used to sort the result-set in ascending or descending order. It 2 min read
- java.nio.ByteOrder Class in Java ByteOrder is a class from java.nio package. In general Byte Order mean the enumeration of ByteOrder. In java there are primitive data types like int, char, float, double are there which will store there data in the main memory in some number of bytes.For example, a character or a short integer occup 3 min read
- AngularJS orderBy Filter An orderBy Filter in AngularJS is used to sort the given array to the specific order. The default order of sorting the string is in alphabetical order whereas the numbers are numerically sorted. By default, all the items are sorted in ascending order, if the ordering sequence is not specified. Synta 4 min read
- ORDER BY Statement in MariaDB In MariaDB, the ORDER BY clause is an important component for organizing the result set of a query in a certain order. Being aware of the complexities of ORDER BY can significantly improve your capacity to get and evaluate data effectively, regardless of whether you're working with one or more colum 3 min read
- Advance Java
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

Spring JPA order by query
Table of Contents

1. Overview
2.1 static sorting, 2.2 dynamic sorting based on parameters, 2.3 order by with jpaspecificationexecutor, 3. conclusion, was this post helpful.
This article is about Spring JPA order by the query, we will explain here soring data with ascending and descending order using spring JPA. While working with relational database Order By is clause using that we can perform sorting the records, the order can be either ascending or descending based on our application requirement we can apply the order, another important is that we can apply to order in a single column or multiple columns in multiple columns both columns might be in the same order or each column has different order, in the below examples we have tried to explain the possibilities of sorting data:
I like to explain that, If we do not specify any order then database by default will not apply for any order. It might be possible that if we execute the same query without order it may return the result in different seqence both the times, Database may return records in any sequence it will not give any guaranty so we working with paging that time we must specify the order, When we are working with paging and do not specify the order then it might be possible that when we move to another page we may get the same records which already been seen in previous pages.
2. Sorting/Order by query examples
Here are examples of static order that we can write in a query or using method names, Order with method name as per spring boot JPA standards :
Order with the query as like normal SQL standers.
Here we have passed that sort as parameters, no need to write order clause in the query. Just pass Sort in as argument other things will be handled by spring JPA. Here we have tried to explain all the possible combinations related to sorting like: single column, multiple columns sorting with ascending and descending orders. We have also given an example related to order by length or we can any aggregate function as per our needs.
Here is an example related to how to pass an order with the dynamic query. Using JpaSpecificationExecutor we can generate a dynamic query, Here we have explained dynamic query with more details.
SQL Query: select * from employee where employeeName=? order by joinedDate desc
In the above examples, we learn different-different examples spring JPA Sorting/Order static as well as dynamic options as well. Ordering may also reduce the query performance we should only apply for the order when we need to show the records in particular order.
4. References
- Spring JPA documentation

Related Articles

Spring boot Scala Example

Spring data JPA where clause

Spring boot disable Whitelabel Error Page
Leave a reply cancel reply.
Your email address will not be published. Required fields are marked *
- More Networks
Maintaining List Order in Jpa
- Dec 29, 2023
If you want to maintain the order of a list of objects retrieve from the database using hibernate/jpa, use the @OrderColumn annotation. An additional column will be created in the database (if ddl is set to update) that will keep track of the order/position of an item in a list.
CAVEAT: If you change the position of an item in a list OR delete an item besides the last one, it will cause a multi update across the list to update all other positions. Be aware of this intensive operation for large lists.
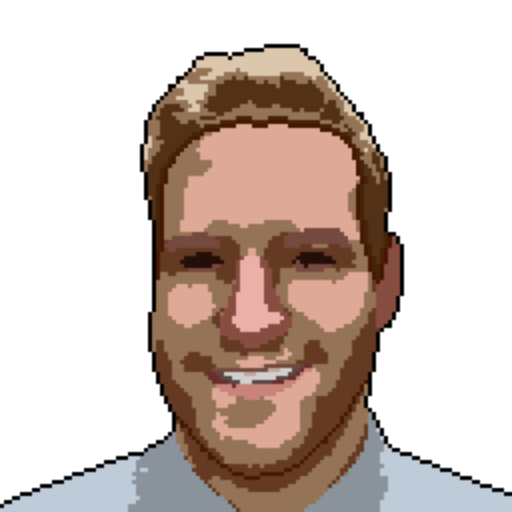
Mark - Creator of QuietShark.com
Welcome! QuietShark.com …
- # back end development (1)
- # batch processing (9)
- # command line (5)
- # database (2)
- # devops (2)
- # front end development (4)
- # hatch (2)
- # javascript (1)
- # kubernetes (6)
- # programming (5)
- # python (3)
- # security (2)
- # spring (2)
- # tooling (1)
Recommended
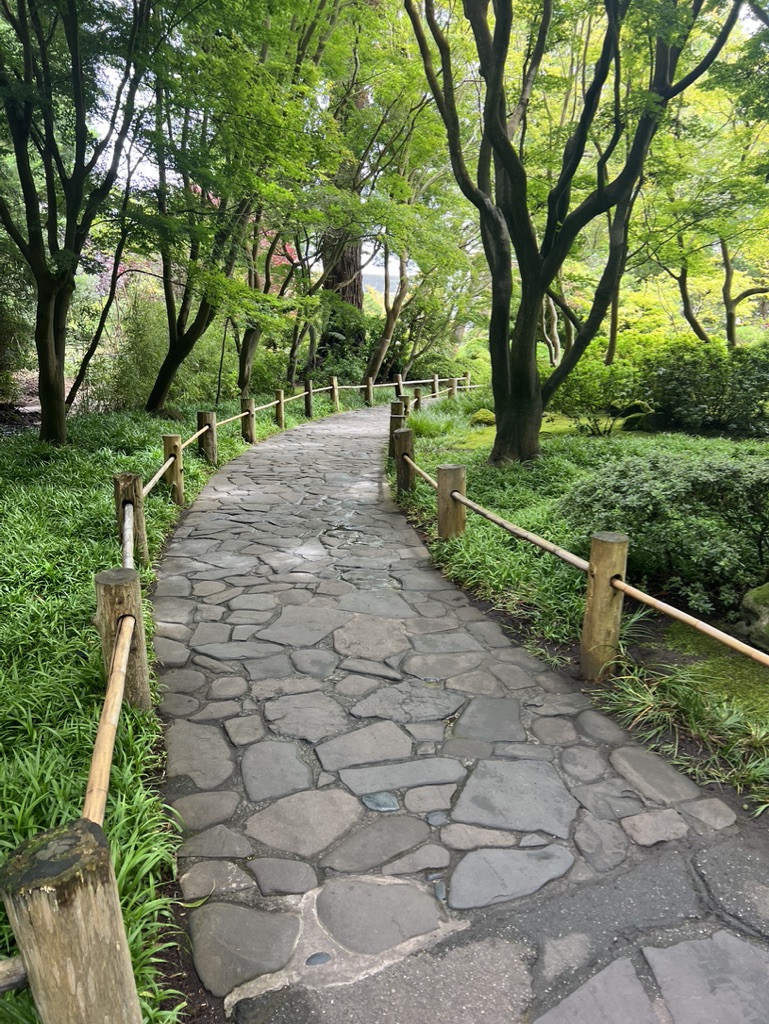
Walrus Operator
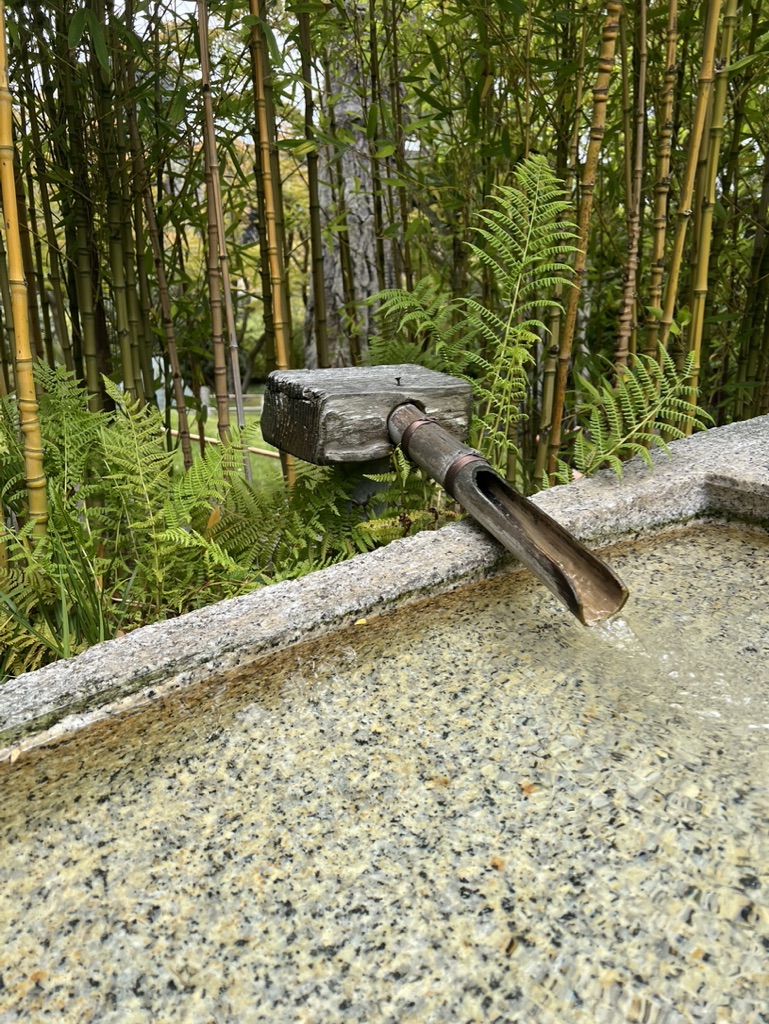
Managing Hatch Dependencies in VS Code
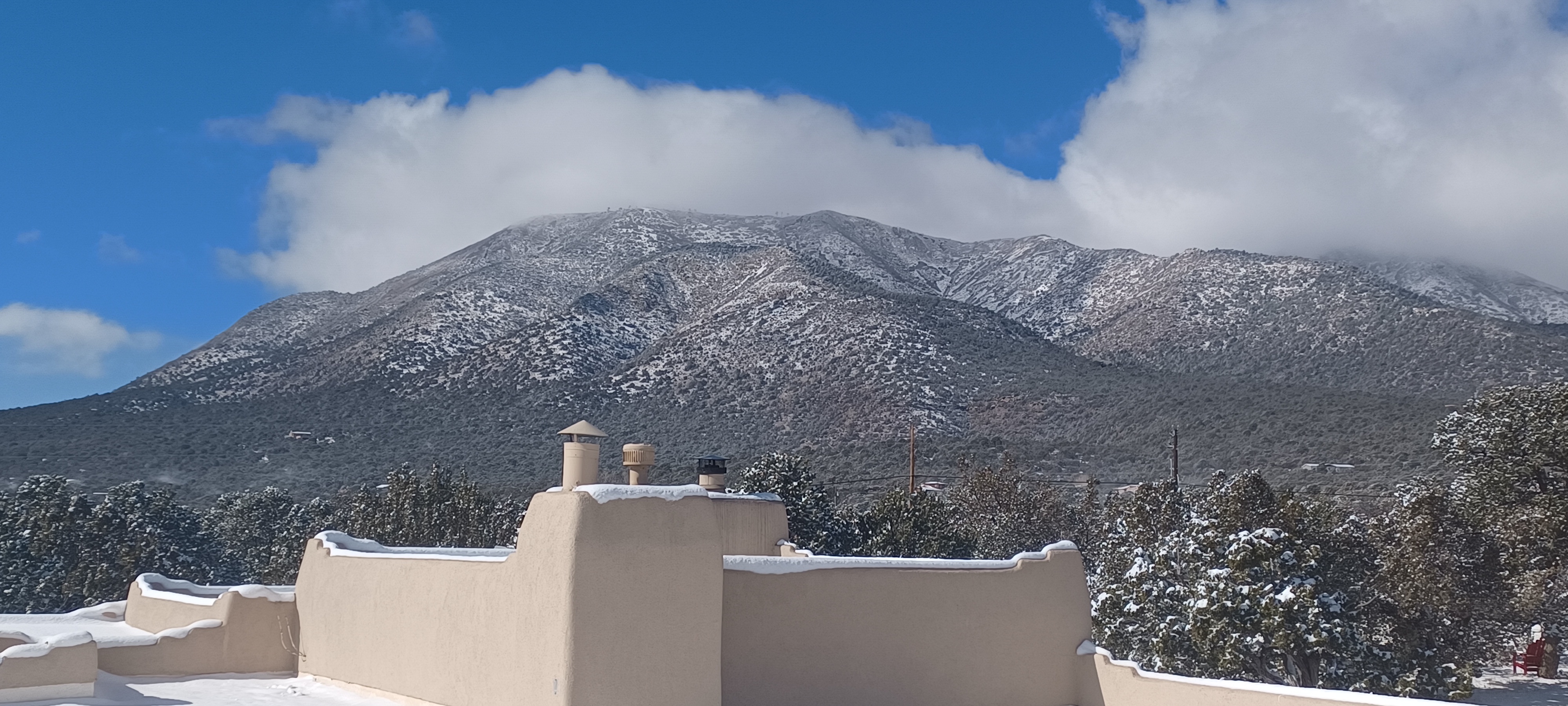
Hatch - a great python project management tool
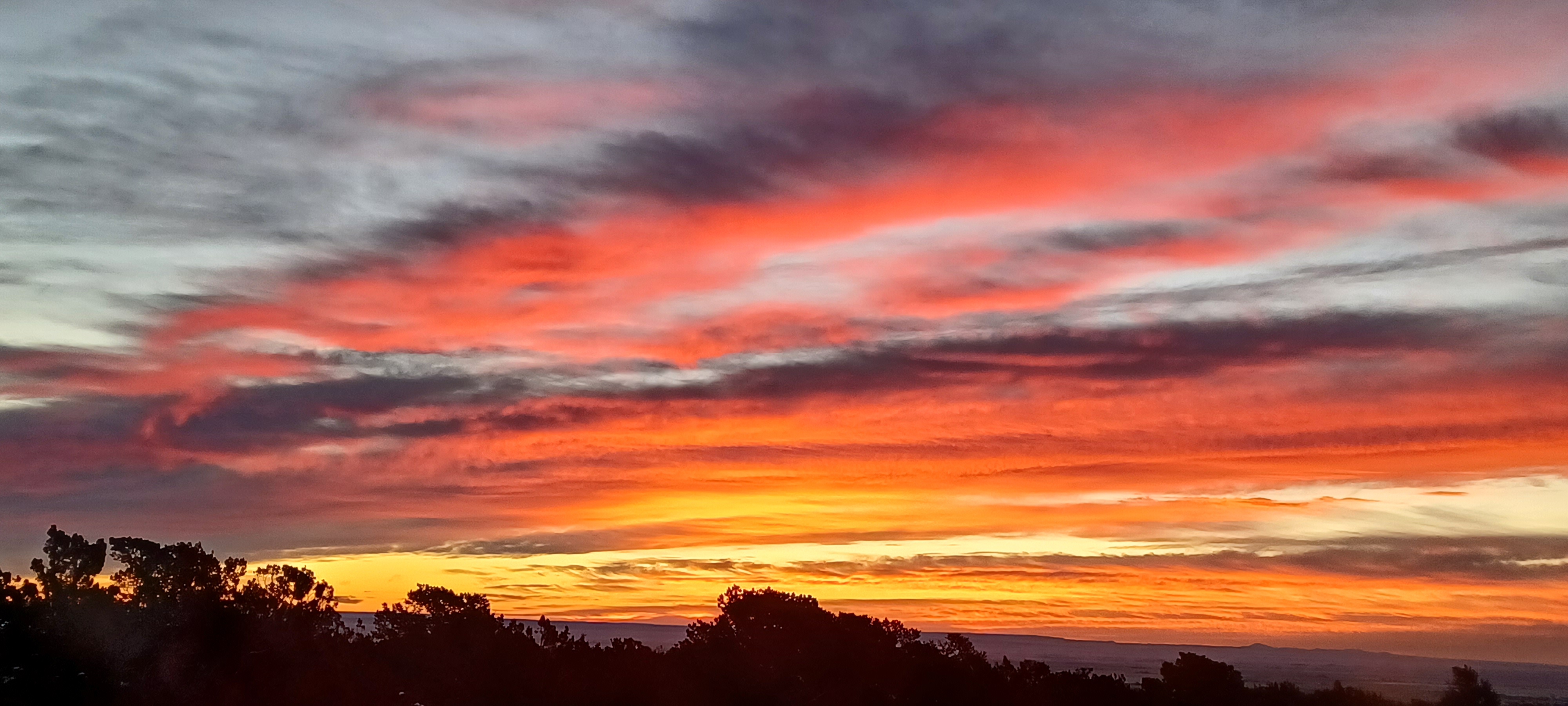
- Send Message
- Core Java Tutorials
- Java EE Tutorials
- Java Swing Tutorials
- Spring Framework Tutorials
- Unit Testing
- Build Tools
- Misc Tutorials
- Address.java
- Employee.java
- ExampleMain.java
- TableMappingMain.java
- persistence.xml

IMAGES
VIDEO
COMMENTS
For your method signature to work as intended with Spring Data JPA, you should include the "all" keyword, like so: List<StudentEntity> findAllByOrderByIdAsc();. Adding a return type and removing the redundant public modifier is also a good idea ;) ... ascending order or descending order by using the value of the id field. Code: public interface ...
How to use order by in JPA Repository. We can use order by in the JPA repository by using the OrderBy keyword in the finder methods of that JPA repository of Spring Data JPA. We n
Step 7: Once the project is completed, run the application.It will then show the Employee name and salary by the descending order as output. Refer the below output image for the better understanding of the concept. By the following the above steps of the article, developers can gain the soild understanding of the how to effectively use the ORDER BY clause in their JPA applications.
In the above examples, we learn different-different examples spring JPA Sorting/Order static as well as dynamic options as well. Ordering may also reduce the query performance we should only apply for the order when we need to show the records in particular order. 4. References. Spring JPA documentation
To apply JPA query hints to the queries declared in your repository interface, you can use the @QueryHints annotation. It takes an array of JPA @QueryHint annotations plus a boolean flag to potentially disable the hints applied to the additional count query triggered when applying pagination, as shown in the following example:
As opposed to simple JQL, the JPA Criteria Query Object API forces an explicit order direction in the query. Notice in the last line of this code snippet that the criteriaBuilder object specifies the sorting order to be ascending by calling its asc method. When the above code is executed, JPA generates the SQL query shown below.
JPA ORDER BY Clause. The ORDER BY clause is used to sort the data and arrange them either in ascending or descending order. The CriteriaQuery interface provides orderBy() method to define the type of ordering. ORDER BY Example. Here, we will perform several ORDER BY operations on student table. Let us assume the table contains the following ...
jpa If you want to maintain the order of a list of objects retrieve from the database using hibernate/jpa, use the @OrderColumn annotation. An additional column will be created in the database (if ddl is set to update) that will keep track of the order/position of an item in a list.
You can save the order of the elements in a java.util.List. In JPA 2.0, There is the good way to save the order of element by using @OrderColumn annotation. For the details, you can refer this link Order Column (JPA 2.0)
The annotation @OrderBy Specifies the ordering of the elements of a collection valued association or element collection at the point when the association or collection is retrieved.. This annotation can be used with @ElementCollection or @OneToMany/@ManyToMany relationships.. When @OrderBy used with @ElementCollection. If the collection is of basic type, then ordering will be by the value of ...